python devtools
Python's missing debug print command and other development tools.
For more information, see documentation.
Install
Just
pip install devtools[pygments]
pygments
is not required but if it's installed, output will be highlighted and easier to read.
devtools
has no other required dependencies except python 3.6, 3.7, or 3.8.
If you've got python 3.6+ and pip
installed, you're good to go.
Usage
from devtools import debug
whatever = [1, 2, 3]
debug(whatever)
Outputs:
test.py:4 <module>:
whatever: [1, 2, 3] (list)
That's only the tip of the iceberg, for example:
import numpy as np
data = {
'foo': np.array(range(20)),
'bar': {'apple', 'banana', 'carrot', 'grapefruit'},
'spam': [{'a': i, 'b': (i for i in range(3))} for i in range(3)],
'sentence': 'this is just a boring sentence.\n' * 4
}
debug(data)
outputs:
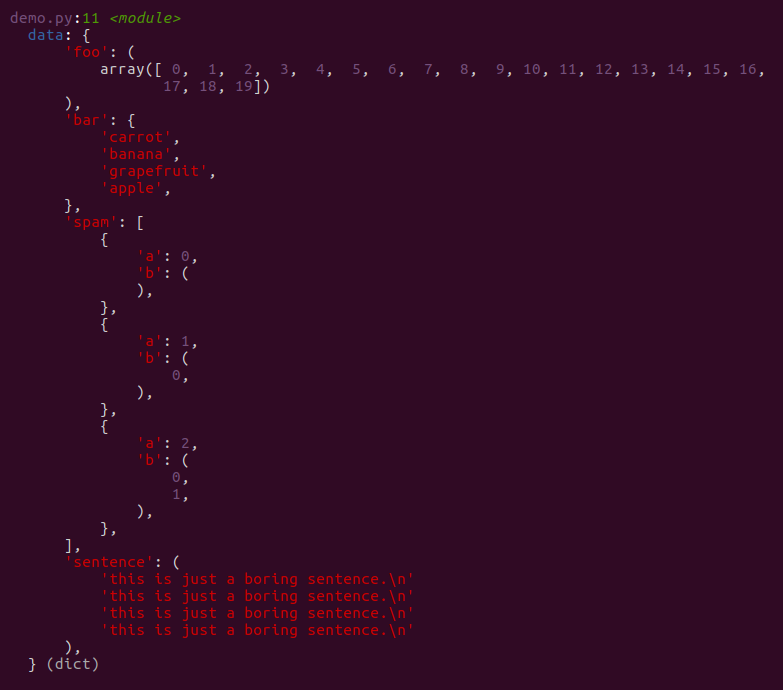
Usage without Import
modify /usr/lib/python3.8/sitecustomize.py
making debug
available in any python 3.8 code
# add devtools debug to builtins
try:
from devtools import debug
except ImportError:
pass
else:
__builtins__['debug'] = debug
GitHub
GitHub - samuelcolvin/python-devtools: Dev tools for python
Dev tools for python. Contribute to samuelcolvin/python-devtools development by creating an account on GitHub.